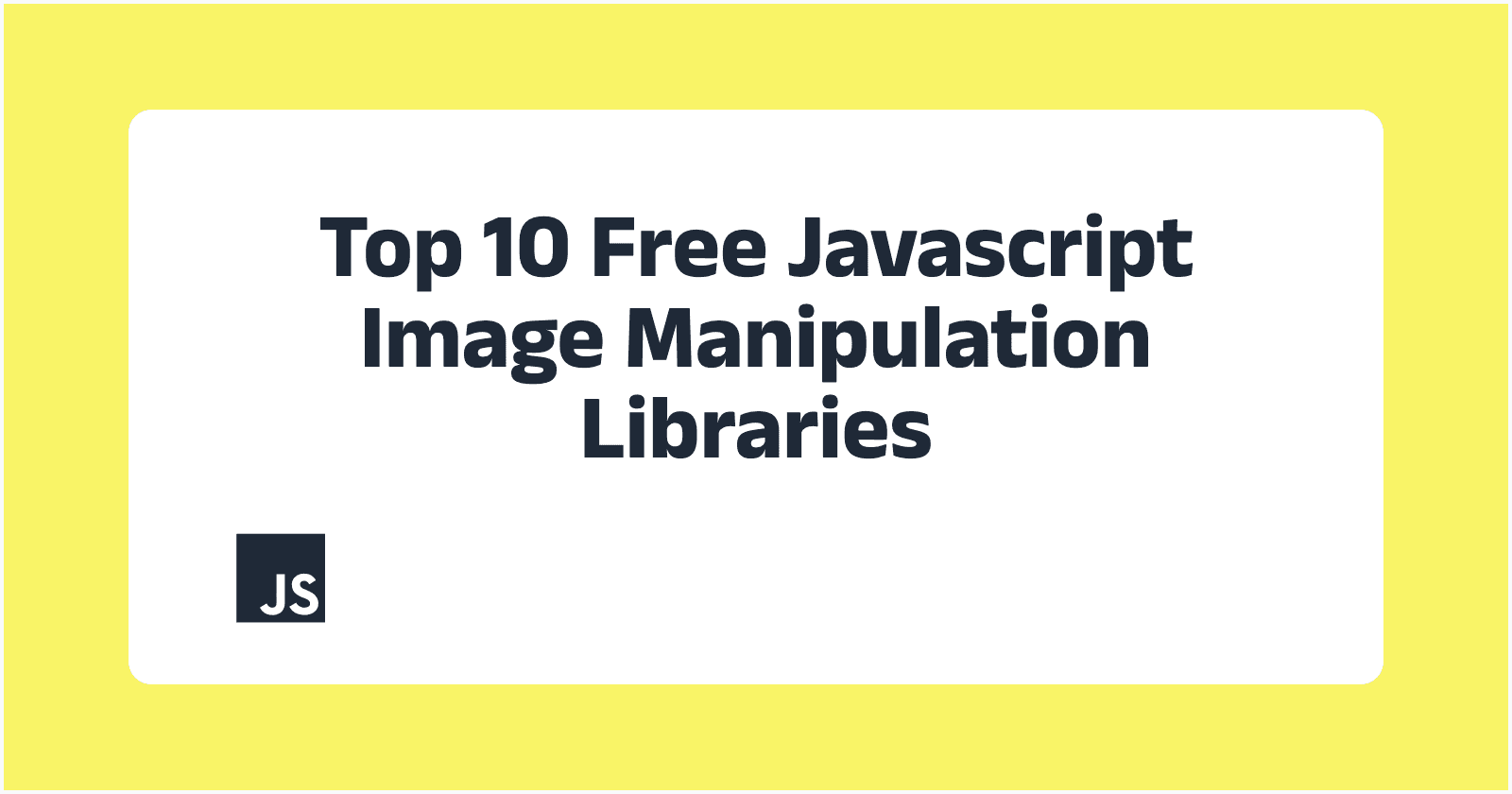
E
Endru Reza
Posted on Nov 5• Originally published at telingadigital.id
Top 10 Free Javascript Image Manipulation Libraries
1. Sharp
Sharp is your typical Node-API module to convert large images in common formats to smaller like JPEG, PNG, WebP, GIF and AVIF with high speed. So long as the system has Node-API v9 and Node.js version ^18.17.0 it will do. It also works on Deno and Bun.
The resizing takes faster time due to its use of lipvips. Sharp can do image resizing, rotation, extraction, compositing and gamma correction.
How to install
bash1# Use your favorite package manager 2bun add sharp
How to use
js1const sharp = require('sharp') 2 3# Callback use 4sharp(inputBuffer) 5 .resize(320, 240) 6 .toFile('output.webp', (err, info) => { ... }); 7 8# Promise use 9sharp('input.jpg') 10 .rotate() 11 .resize(200) 12 .jpeg({ mozjpeg: true }) 13 .toBuffer() 14 .then( data => { ... }) 15 .catch( err => { ... }); 16 17# Async/await use 18const semiTransparentRedPng = await sharp({ 19 create: { 20 width: 48, 21 height: 48, 22 channels: 4, 23 background: { r: 255, g: 0, b: 0, alpha: 0.5 } 24 } 25}) 26 .png() 27 .toBuffer(); 28 29# Stream use 30const roundedCorners = Buffer.from( 31 '<svg><rect x="0" y="0" width="200" height="200" rx="50" ry="50"/></svg>' 32); 33 34const roundedCornerResizer = 35 sharp() 36 .resize(200, 200) 37 .composite([{ 38 input: roundedCorners, 39 blend: 'dest-in' 40 }]) 41 .png(); 42 43readableStream 44 .pipe(roundedCornerResizer) 45 .pipe(writableStream);
Features
- Resizing Images
- Compositing Images
- Image Operation
- Color Manipulation
- Channel Manipulation
2. Cropper.js
Cropper.js is your typical library when you want to crop current image.
How To Install
bash1# Use your favorite package manager 2bun add cropperjs
How To Use
js1// import 'cropperjs/dist/cropper.css'; 2import Cropper from 'cropperjs'; 3 4const image = document.getElementById('image'); 5const cropper = new Cropper(image, { 6 aspectRatio: 16 / 9, 7 crop(event) { 8 console.log(event.detail.x); 9 console.log(event.detail.y); 10 console.log(event.detail.width); 11 console.log(event.detail.height); 12 console.log(event.detail.rotate); 13 console.log(event.detail.scaleX); 14 console.log(event.detail.scaleY); 15 }, 16});
Features
- 39 Options
- 27 Methods
- 6 events
- Touch (mobile)
- Zooming
- Rotating
- Scaling (flipping)
- Multiple croppers
- Cropping on canvas
- Cropping an image on the browser-side by canvas
- Translating Exif Orientation information
- Cross-browser
3. Jimp
Jimp is your typical library when you want to load and manipulate image at the same time in the the browser and Node.js easily. Just for a spoiler, since it's built on Javascript implementation of image formats, it's not optimized for performance and may allocate a lot of memory.
How To Install
bash1bun add jimp
How To Use
js1import { Jimp } from "jimp"; 2 3const image = await Jimp.read("text/image.png"); 4image.resize({ width: 100 }); 5await image.write("test/output.png");
Another Way to use
js1# With hosted file 2 3import { Jimp } from "jimp"; 4 5// Read a file hosted on the same domain 6const image1 = await Jimp.read("/some/url"); 7 8// Read a file hosted on a different domain 9const image2 = await Jimp.read("https://some.other.domain/some/url");
js1# With uploaded file 2 3function handleFile(e: React.ChangeEvent<HTMLInputElement>) { 4 const reader = new FileReader(); 5 6 reader.onload = async (e) => { 7 const image = await Jimp.fromBuffer(e.target?.result); 8 9 image.greyscale(); 10 11 const base64 = await image.getBase64("image/jpeg"); 12 }; 13 14 reader.readAsArrayBuffer(e.target.files[0]); 15} 16 17input.addEventListener("change", handleFile);
js1# Using Canvas 2 3const canvas = document.getElementById("my-canvas"); 4const ctx = canvas.getContext("2d"); 5 6// Load the canvas into a Jimp instance 7const image = await Jimp.fromBitmap( 8 ctx.getImageData(0, 0, canvas.width, canvas.height) 9); 10 11// Manipulate the image 12image.greyscale(); 13 14const imageData = new ImageData( 15 new Uint8ClampedArray(image.bitmap.data), 16 image.bitmap.width, 17 image.bitmap.height 18); 19 20// Write back to the canvas 21ctx.putImageData(imageData, 0, 0);
js1# Using web workers 2 3import { Jimp, loadFont } from "jimp"; 4 5// eslint-disable-next-line @typescript-eslint/no-explicit-any 6const ctx: Worker = self as any; 7 8ctx.addEventListener("message", async (e) => { 9 // Initialize Jimp 10 const image = await Jimp.fromBuffer(e.data.image); 11 const options = e.data.options; 12 13 // Manipulate the image 14 if (options.blur) { 15 image.blur(options.blur); 16 } 17 18 // Return the result 19 ctx.postMessage({ base64: await image.getBase64("image/png") }); 20}); 21 22// then 23const fileData: ArrayBuffer = new ArrayBuffer(); // Your image data 24const worker = new Worker(new URL("./jimp.worker.ts", import.meta.url), { 25 type: "module", 26}); 27 28worker.postMessage({ 29 image: fileData, 30 options: { 31 blur: 8 32 }, 33}); 34 35worker.addEventListener("message", (e) => { 36 setOutput(e.data.base64); 37 setIsLoading(false); 38});
Features
- Support PNG, JPEG, BMP, GIF and TIFF.
- Support resize, crop, apply filters and more.
- You can create your own plugins to add new image manipulation methods or formats
- It's pure javascript. So long as the system use javascript, you could use this library
4. Merge Images
Merge images is your typical library when you want to join multiple images to create a more informative images. Or, you just want to merge images.
How To Install
bash1# Use your favorite package manager 2bun add merge-images
How To Use
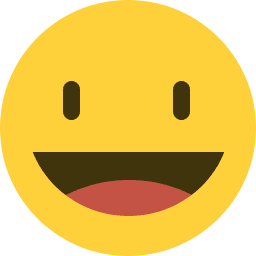
js1import mergeImages from 'merge-images'; 2 3mergeImages(['/body.png', '/eyes.png', '/mouth.png']) 4 .then(b64 => document.querySelector('img').src = b64); 5 // data:image/png;base64,iVBORw0KGgoAA...
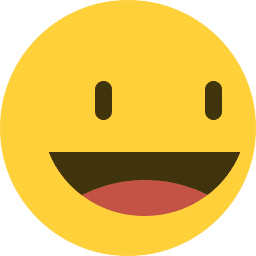
js1# Positioning 2 3mergeImages([ 4 { src: 'body.png', x: 0, y: 0 }, 5 { src: 'eyes.png', x: 32, y: 0 }, 6 { src: 'mouth.png', x: 16, y: 0 } 7]) 8 .then(b64 => ...); 9 // data:image/png;base64,iVBORw0KGgoAA...
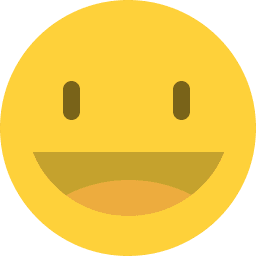
js1# Opacity 2 3mergeImages([ 4 { src: 'body.png' }, 5 { src: 'eyes.png', opacity: 0.7 }, 6 { src: 'mouth.png', opacity: 0.3 } 7]) 8 .then(b64 => ...); 9 // data:image/png;base64,iVBORw0KGgoAA...
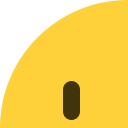
js1# Dimensions 2 3mergeImages(['/body.png', '/eyes.png', '/mouth.png'], { 4 width: 128, 5 height: 128 6}) 7 .then(b64 => ...); 8 // data:image/png;base64,iVBORw0KGgoAA...
js1# Nodejs Usage requires node-canvas 2 3const mergeImages = require('merge-images'); 4const { Canvas, Image } = require('canvas'); 5 6mergeImages(['./body.png', './eyes.png', './mouth.png'], { 7 Canvas: Canvas, 8 Image: Image 9}) 10 .then(b64 => ...); 11 // data:image/png;base64,iVBORw0KGgoAA...
Features
- Merging multiple images
- Positioning each image
- Set each image opacity
- Set each image dimension
5. IPX
IPX is your typical library when you want a high-performance, secure and easy-to-use image optimizer. This library is powered by Sharp and svgo.
How To Install
bash1# Use your own favorite package manager 2 3npx ipx serve --dir ./
How To Use
js1import { createIPX, ipxFSStorage, ipxHttpStorage } from "ipx"; 2 3const ipx = createIPX({ 4 storage: ipxFSStorage({ dir: "./public" }), 5 httpStorage: ipxHttpStorage({ domains: ["picsum.photos"] }), 6});
Features
Since it's powered by sharp, whatever Sharp could do, IPX could do too.
6. Smartcrop.js
Smartcrop would be the best choice if you really want to smart and precision cropper. The developer is even working on advanced version of this library based on machine learning.
How To Install
bash1# Use your favorite package manager 2 3bun add smartcrop
How To Use
js1// you pass in an image as well as the width & height of the crop you 2// want to optimize. 3smartcrop.crop(image, { width: 100, height: 100 }).then(function(result) { 4 console.log(result); 5}); 6 7# Output 8// smartcrop will output you its best guess for a crop 9// you can now use this data to crop the image. 10{topCrop: {x: 300, y: 200, height: 200, width: 200}}
Features
The most feature that you could find with this package is that this library implements content aware on their image cropping.
7. Satori
Satori is a library made by Vercel to convert HTML and CSS to SVG easily.
How To Install
bash1# Use your favorite package manager 2 3bun add satori
How To Use
js1// api.jsx 2import satori from 'satori' 3 4const svg = await satori( 5 <div style={{ color: 'black' }}>hello, world</div>, 6 { 7 width: 600, 8 height: 400, 9 fonts: [ 10 { 11 name: 'Roboto', 12 // Use `fs` (Node.js only) or `fetch` to read the font as Buffer/ArrayBuffer and provide `data` here. 13 data: robotoArrayBuffer, 14 weight: 400, 15 style: 'normal', 16 }, 17 ], 18 }, 19) 20 21# Output 22 23'<svg ...><path d="..." fill="black"></path></svg>'
Features
- Support JSX, Non-JSX, HTML, CSS, Fonts, Emojis, WASM.
8. Pintura
Pintura is a really powerful Javascript image editor that could be integrated with every stack.
How To Install
bash1# Use your favorite package manager 2 3bun add @pqina/pintura
How To Use
js1import '@pqina/pintura/pintura.css'; 2import { openDefaultEditor } from '@pqina/pintura'; 3 4openDefaultEditor({ 5 src: 'image.jpeg' 6});
Another Use
jsx1# React Example 2 3import { PinturaEditor } from '@pqina/react-pintura'; 4import { getEditorDefaults } from '@pqina/pintura'; 5 6import '@pqina/pintura/pintura.css'; 7 8function App() { 9 const [result, setResult] = useState(); 10 11 return ( 12 <div> 13 <PinturaEditor 14 {...getEditorDefaults()} 15 src="my-image.jpeg" 16 onProcess={(res) => setResult(URL.createObjectURL(res.dest))} 17 /> 18 19 {result && <img src={result} alt="" />} 20 </div> 21 ); 22} 23 24export default App;
Features
- Support every stack
- Crop, rotate, resize, filter, annotate, adjust colors and much more
9. ToastUI Image Editor
ToastUI Image Editor is a full featured image editor using HTML5 Canvas. It's easy to use and provides lot of filters.
How To Install
bash1# Use your favorite package manager 2 3bun add tui-image-editor
How To Use
js1const ImageEditor = require('tui-image-editor'); 2const instance = new ImageEditor(document.querySelector('#tui-image-editor'), { 3 cssMaxWidth: 700, 4 cssMaxHeight: 500, 5 selectionStyle: { 6 cornerSize: 20, 7 rotatingPointOffset: 70, 8 }, 9});
Features
- Load image to canvas
- Undo/Redo (with shortcut)
- Crop
- Flip
- Rotation
- Resize
- Free drawing
- Line drawing
- Shape
- Icon
- Text
- Mask Filter
- Image Filter
10. Pica
Pica would be a good choice if you want to resize images in browser without pixelation and doing it fast. It involves best available technologies such as webworkers, webassembly, createImageBitmap and pure JS.
How To Install
bash1# Use your favorite package manager 2 3bun add pica
How To Use
js1const pica = require('pica')(); 2 3// Resize from Canvas/Image to another Canvas 4pica.resize(from, to) 5 .then(result => console.log('resize done!')); 6 7// Resize & convert to blob 8pica.resize(from, to) 9 .then(result => pica.toBlob(result, 'image/jpeg', 0.90)) 10 .then(blob => console.log('resized to canvas & created blob!'));
Features
- Reduce upload size for large images, saving upload time
- Saves server resources on image processing
- Generate thumbnails in browser
11 min read